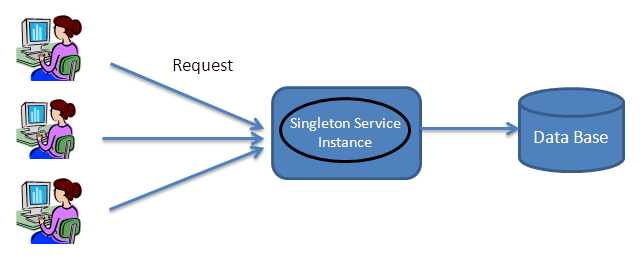
Implementing the Singleton Design Pattern in JavaScript
What is the Singleton design pattern?
Implementing the Singleton pattern
The Singleton design pattern is a creational pattern that states that one and only one instance of a class would persist in the memory during the application's life cycle. In other words, this design pattern restricts instantiation of a class to just one object. As far as JavaScript is concerned, a singleton is an instance that is created just once — any repeated calls to the constructor would always fetch the same instance.
An easy way to implement the Singleton design pattern in JavaScript is by using an object literal as shown below.
var singleton = { method1: function () { //Write your usual code here }, method2: function () { //Write your usual code here } };
Here's another code snippet that illustrates how you can implement the Singleton pattern in JavaScript.
var Singleton = (function(){ function Singleton() { //Write your usual code here } var instance; return { getInstance: function(){ if (null == instance) { instance = new Singleton(); instance.constructor = null; // Note how the constructor is hidden to prevent instantiation } return instance; //return the singleton instance } }; })();
Refer to the code snippet given above. Note how a check is made to see if the instance is null. If the instance is null, a new instance is created and returned by suppressing/hiding the constructor. If the instance is non-null, the instance is returned. This approach is also known as the module pattern. As you can see, you can encapsulate the private members that belong to a particular instance using closures.
Now that we have implemented our Singleton class, the following code snippet can be used to invoke the function.
var justOneInstance = Singleton.getInstance();
The GOF states: "The Singleton Pattern limits the number of instances of a particular object to just one. This single instance is called the singleton. Singletons are useful in situations where system-wide actions need to be coordinated from a single central place. An example is a database connection pool. The pool manages the creation, destruction, and lifetime of all database connections for the entire application ensuring that no connections are 'lost'."
Source: HTML Goodies